Create a Login Authentication Module
This page shows how to create a reusable login authentication module that allows users to authenticate through email and password credentials stored in an SQL database.
Prerequisites
- A package that has already been created. For more information, see Package and query modules tutorials.
- A datasource connected to that workspace with user authentication credentials.
Configure package
Follow these steps to set up modules within the package.
- Create a new query module that allows you to verify whether the user's email exists within the database.
Create inputs from the right-side property pane to pass the email dynamically from the JS module. Use the inputs
in the query, like:
//findUserByEmail
SELECT * FROM public.auth_users WHERE email = {{inputs.email}};
- Create a new Datasource query for updating the last login timestamp:
Create inputs from the right-side property pane to pass the user ID dynamically from the JS module. Use the inputs
in the query, like this:
//updateLogin
UPDATE public.auth_users
SET last_login = {{new Date().toISOString()}}
WHERE id = {{ inputs.id }};
- Create a new JS module to handle the authentication process:
This JS module verifies user credentials, updates the last login timestamp upon successful authentication, generates a session token, and provides feedback through alerts for user login attempts.
You can use the Appsmith Object and Functions within the JS module code, which can be executed in the App, like:
export default {
// The login function attempts to authenticate a user
login: async (email, passwordHash) => {
// Retrieve user data from the database based on the provided email
const [user] = await findUserByEmail.run({ email });
// Check if a user with the provided email exists and the password hash matches
if (user && passwordHash === user?.password_hash) {
// Generate a token using the current timestamp and email, and store it
await storeValue('token', btoa(new Date().toISOString() + email));
// Update the last login timestamp for the user in the database
await updateLogin.run({ id: user.id });
// Show a success alert indicating successful login
await showAlert('Login Success', 'success');
// Navigate to the 'App' page in the same window after successful login
await navigateTo('App', {}, 'SAME_WINDOW');
} else {
// Show an error alert indicating login failure
await showAlert('Login Failed: Invalid email or password', 'error');
}
}
}
-
To pass data from the App to JS modules, you can pass it by calling the respective function with the necessary parameters, like
login(hello@email.com, password@123)
. -
To pass data from JS modules to queries, you can pass parameters at runtime using
run()
, like{{ updateLogin.run({ id: user.id }) }}
- Publish the package.
Integrate the Login Module
Follow these steps to use the login authentication module within your application:
-
Open your App from the homepage and ensure that both the app and modules share the same workspace.
-
Create a simple login form using widgets such as Text and Input fields, tailored to meet your specific requirements.
-
Select the JS tab on the Entity Explorer, add the Login JS module, and pass login form data into function parameters for authentication:
- email:
{{emailInput.text}}
- passwordHash:
{{passwordInput.text}}
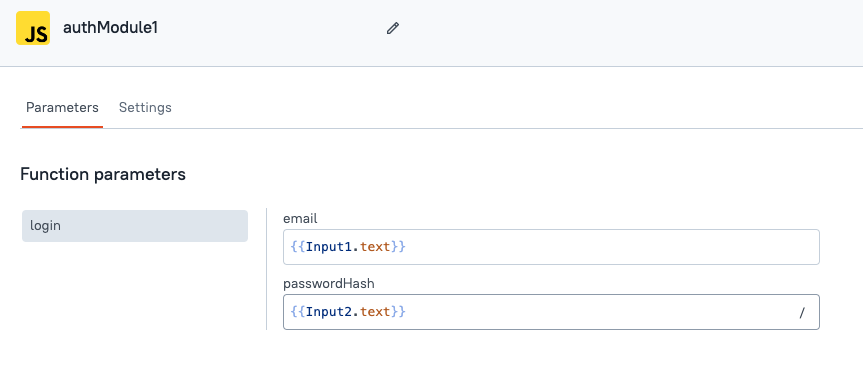
- Set the onClick event of the Submit/Login button to run the JS module.
When the button is clicked, the JS module verifies whether the user's email exists in the database. If the email and password match, it redirects the user to a new page.